Members
animationAdded :Event
Type:
- Default Value:
- new Event()
- Source:
Example
model.activeAnimations.animationAdded.addEventListener(function(model, animation) {
console.log('Animation added: ' + animation.name);
});
animationRemoved :Event
Type:
- Default Value:
- new Event()
- Source:
Example
model.activeAnimations.animationRemoved.addEventListener(function(model, animation) {
console.log('Animation removed: ' + animation.name);
});
boundingVolume :Object
For best rendering performance, use the tightest possible bounding volume. Although
undefined
is allowed, always try to provide a bounding volume to
allow the tightest possible near and far planes to be computed for the scene, and
minimize the number of frustums needed.
Type:
- Object
- Default Value:
- undefined
- Source:
- See:
-
- DrawCommand#debugShowBoundingVolume
castShadows :Boolean
Type:
- Boolean
- Default Value:
- false
- Source:
clockTrackedDataSource :DataSource
Type:
- Source:
count :Number
Type:
- Number
- Default Value:
- undefined
- Source:
cull :Boolean
true
, the renderer frustum and horizon culls the command based on its DrawCommand#boundingVolume
.
If the command was already culled, set this to false
for a performance improvement.
Type:
- Boolean
- Default Value:
- true
- Source:
czm_currentFrustum
x
) and the far distance (y
)
of the frustum defined by the camera. This is the individual
frustum used for multi-frustum rendering.
Example
// GLSL declaration
uniform vec2 czm_currentFrustum;
// Example
float frustumLength = czm_currentFrustum.y - czm_currentFrustum.x;
czm_encodedCameraPositionMCHigh
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_encodedCameraPositionMCHigh;
czm_encodedCameraPositionMCLow
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_encodedCameraPositionMCLow;
czm_entireFrustum
x
) and the far distance (y
)
of the frustum defined by the camera. This is the largest possible frustum, not an individual
frustum used for multi-frustum rendering.
Example
// GLSL declaration
uniform vec2 czm_entireFrustum;
// Example
float frustumLength = czm_entireFrustum.y - czm_entireFrustum.x;
czm_eyeHeight2D
x
) and height squared (y
)
of the eye (camera) in the 2D scene in meters.
- Source:
- See:
czm_fogDensity
- Source:
- See:
-
- czm_fog
czm_frameNumber
- Source:
Example
// GLSL declaration
uniform float czm_frameNumber;
czm_frustumPlanes
- Source:
czm_infiniteProjection
gl_Position
output. An infinite far plane is used
in algorithms like shadow volumes and GPU ray casting with proxy geometry to ensure that triangles
are not clipped by the far plane.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_infiniteProjection;
// Example
gl_Position = czm_infiniteProjection * eyePosition;
czm_inverseModel
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_inverseModel;
// Example
vec4 modelPosition = czm_inverseModel * worldPosition;
czm_inverseModelView
Example
// GLSL declaration
uniform mat4 czm_inverseModelView;
// Example
vec4 modelPosition = czm_inverseModelView * eyePosition;
czm_inverseModelView3D
czm_inverseModelView
, but in 2D and Columbus View it represents the inverse model-view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_inverseModelView3D;
// Example
vec4 modelPosition = czm_inverseModelView3D * eyePosition;
czm_inverseModelViewProjection
gl_Position
output.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_inverseModelViewProjection;
// Example
vec4 modelPosition = czm_inverseModelViewProjection * clipPosition;
czm_inverseNormal
czm_normal
.
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_inverseNormal;
// Example
vec3 normalMC = czm_inverseNormal * normalEC;
czm_inverseNormal3D
czm_normal
.
In 3D mode, this is identical to
czm_inverseNormal
, but in 2D and Columbus View it represents the inverse normal transformation
matrix as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_inverseNormal3D;
// Example
vec3 normalMC = czm_inverseNormal3D * normalEC;
czm_inverseProjection
gl_Position
output.
Example
// GLSL declaration
uniform mat4 czm_inverseProjection;
// Example
vec4 eyePosition = czm_inverseProjection * clipPosition;
czm_inverseView
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_inverseView;
// Example
vec4 worldPosition = czm_inverseView * eyePosition;
czm_inverseView3D
czm_inverseView
, but in 2D and Columbus View it represents the inverse view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
Example
// GLSL declaration
uniform mat4 czm_inverseView3D;
// Example
vec4 worldPosition = czm_inverseView3D * eyePosition;
czm_inverseViewProjection
gl_Position
output.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_inverseViewProjection;
// Example
vec4 worldPosition = czm_inverseViewProjection * clipPosition;
czm_inverseViewRotation
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_inverseViewRotation;
// Example
vec4 worldVector = czm_inverseViewRotation * eyeVector;
czm_inverseViewRotation3D
czm_inverseViewRotation
, but in 2D and Columbus View it represents the inverse view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_inverseViewRotation3D;
// Example
vec4 worldVector = czm_inverseViewRotation3D * eyeVector;
czm_model
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_model;
// Example
vec4 worldPosition = czm_model * modelPosition;
czm_modelView
Positions should be transformed to eye coordinates using
czm_modelView
and
normals should be transformed using czm_normal
.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_modelView;
// Example
vec4 eyePosition = czm_modelView * modelPosition;
// The above is equivalent to, but more efficient than:
vec4 eyePosition = czm_view * czm_model * modelPosition;
czm_modelView3D
czm_modelView
, but in 2D and Columbus View it represents the model-view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
Positions should be transformed to eye coordinates using
czm_modelView3D
and
normals should be transformed using czm_normal3D
.
Example
// GLSL declaration
uniform mat4 czm_modelView3D;
// Example
vec4 eyePosition = czm_modelView3D * modelPosition;
// The above is equivalent to, but more efficient than:
vec4 eyePosition = czm_view3D * czm_model * modelPosition;
czm_modelViewInfiniteProjection
gl_Position
output. The projection matrix places
the far plane at infinity. This is useful in algorithms like shadow volumes and GPU ray casting with
proxy geometry to ensure that triangles are not clipped by the far plane.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_modelViewInfiniteProjection;
// Example
vec4 gl_Position = czm_modelViewInfiniteProjection * modelPosition;
// The above is equivalent to, but more efficient than:
gl_Position = czm_infiniteProjection * czm_view * czm_model * modelPosition;
czm_modelViewProjection
gl_Position
output.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_modelViewProjection;
// Example
vec4 gl_Position = czm_modelViewProjection * modelPosition;
// The above is equivalent to, but more efficient than:
gl_Position = czm_projection * czm_view * czm_model * modelPosition;
czm_modelViewProjectionRelativeToEye
gl_Position
output. This is used in
conjunction with czm_translateRelativeToEye
.
- Source:
- See:
-
- czm_modelViewRelativeToEye
- czm_translateRelativeToEye
- EncodedCartesian3
Example
// GLSL declaration
uniform mat4 czm_modelViewProjectionRelativeToEye;
// Example
attribute vec3 positionHigh;
attribute vec3 positionLow;
void main()
{
vec4 p = czm_translateRelativeToEye(positionHigh, positionLow);
gl_Position = czm_modelViewProjectionRelativeToEye * p;
}
czm_modelViewRelativeToEye
czm_translateRelativeToEye
.
- Source:
- See:
-
- czm_modelViewProjectionRelativeToEye
- czm_translateRelativeToEye
- EncodedCartesian3
Example
// GLSL declaration
uniform mat4 czm_modelViewRelativeToEye;
// Example
attribute vec3 positionHigh;
attribute vec3 positionLow;
void main()
{
vec4 p = czm_translateRelativeToEye(positionHigh, positionLow);
gl_Position = czm_projection * (czm_modelViewRelativeToEye * p);
}
czm_moonDirectionEC
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_moonDirectionEC;
// Example
float diffuse = max(dot(czm_moonDirectionEC, normalEC), 0.0);
czm_morphTime
- Source:
Example
// GLSL declaration
uniform float czm_morphTime;
// Example
vec4 p = czm_columbusViewMorph(position2D, position3D, czm_morphTime);
czm_normal
Positions should be transformed to eye coordinates using
czm_modelView
and
normals should be transformed using czm_normal
.
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_normal;
// Example
vec3 eyeNormal = czm_normal * normal;
czm_normal3D
czm_normal
, but in 2D and Columbus View it represents the normal transformation
matrix as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
Positions should be transformed to eye coordinates using
czm_modelView3D
and
normals should be transformed using czm_normal3D
.
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_normal3D;
// Example
vec3 eyeNormal = czm_normal3D * normal;
czm_pass
- Source:
Example
// GLSL declaration
uniform float czm_pass;
// Example
if ((czm_pass == czm_passTranslucent) && isOpaque())
{
gl_Position *= 0.0; // Cull opaque geometry in the translucent pass
}
czm_projection
gl_Position
output.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_projection;
// Example
gl_Position = czm_projection * eyePosition;
czm_resolutionScale
- Source:
Example
uniform float czm_resolutionScale;
czm_sceneMode
SceneMode
, expressed
as a float.
- Source:
- See:
-
- czm_sceneMode2D
- czm_sceneModeColumbusView
- czm_sceneMode3D
- czm_sceneModeMorphing
Example
// GLSL declaration
uniform float czm_sceneMode;
// Example
if (czm_sceneMode == czm_sceneMode2D)
{
eyeHeightSq = czm_eyeHeight2D.y;
}
czm_sunDirectionEC
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_sunDirectionEC;
// Example
float diffuse = max(dot(czm_sunDirectionEC, normalEC), 0.0);
czm_sunDirectionWC
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_sunDirectionWC;
czm_sunPositionColumbusView
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_sunPositionColumbusView;
czm_sunPositionWC
- Source:
- See:
Example
// GLSL declaration
uniform vec3 czm_sunPositionWC;
czm_temeToPseudoFixed
- Source:
- See:
-
- UniformState#temeToPseudoFixedMatrix
- Transforms.computeTemeToPseudoFixedMatrix
Example
// GLSL declaration
uniform mat3 czm_temeToPseudoFixed;
// Example
vec3 pseudoFixed = czm_temeToPseudoFixed * teme;
czm_view
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_view;
// Example
vec4 eyePosition = czm_view * worldPosition;
czm_view3D
czm_view
, but in 2D and Columbus View it represents the view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_view3D;
// Example
vec4 eyePosition3D = czm_view3D * worldPosition3D;
czm_viewerPositionWC
- Source:
Example
// GLSL declaration
uniform vec3 czm_viewerPositionWC;
czm_viewport
x
, y
, width
,
and height
properties in an vec4
's x
, y
, z
,
and w
components, respectively.
- Source:
- See:
-
- Context#getViewport
Example
// GLSL declaration
uniform vec4 czm_viewport;
// Scale the window coordinate components to [0, 1] by dividing
// by the viewport's width and height.
vec2 v = gl_FragCoord.xy / czm_viewport.zw;
czm_viewportOrthographic
gl_Position
output.
This transform is useful when a vertex shader inputs or manipulates window coordinates as done by
BillboardCollection
.
Do not confuse
czm_viewportTransformation
with czm_viewportOrthographic
.
The former transforms from normalized device coordinates to window coordinates; the later transforms
from window coordinates to clip coordinates, and is often used to assign to gl_Position
.
- Source:
- See:
-
- UniformState#viewportOrthographic
- czm_viewport
- czm_viewportTransformation
- BillboardCollection
Example
// GLSL declaration
uniform mat4 czm_viewportOrthographic;
// Example
gl_Position = czm_viewportOrthographic * vec4(windowPosition, 0.0, 1.0);
czm_viewportTransformation
near = 0
and far = 1
.
This transform is useful when there is a need to manipulate window coordinates in a vertex shader as done by
BillboardCollection
. In many cases,
this matrix will not be used directly; instead, czm_modelToWindowCoordinates
will be used to transform directly from model to window coordinates.
Do not confuse
czm_viewportTransformation
with czm_viewportOrthographic
.
The former transforms from normalized device coordinates to window coordinates; the later transforms
from window coordinates to clip coordinates, and is often used to assign to gl_Position
.
- Source:
- See:
-
- UniformState#viewportTransformation
- czm_viewport
- czm_viewportOrthographic
- czm_modelToWindowCoordinates
- BillboardCollection
Example
// GLSL declaration
uniform mat4 czm_viewportTransformation;
// Use czm_viewportTransformation as part of the
// transform from model to window coordinates.
vec4 q = czm_modelViewProjection * positionMC; // model to clip coordinates
q.xyz /= q.w; // clip to normalized device coordinates (ndc)
q.xyz = (czm_viewportTransformation * vec4(q.xyz, 1.0)).xyz; // ndc to window coordinates
czm_viewProjection
gl_Position
output.
- Source:
- See:
Example
// GLSL declaration
uniform mat4 czm_viewProjection;
// Example
vec4 gl_Position = czm_viewProjection * czm_model * modelPosition;
// The above is equivalent to, but more efficient than:
gl_Position = czm_projection * czm_view * czm_model * modelPosition;
czm_viewRotation
- Source:
- See:
Example
// GLSL declaration
uniform mat3 czm_viewRotation;
// Example
vec3 eyeVector = czm_viewRotation * worldVector;
czm_viewRotation3D
czm_viewRotation
, but in 2D and Columbus View it represents the view matrix
as if the camera were at an equivalent location in 3D mode. This is useful for lighting
2D and Columbus View in the same way that 3D is lit.
Example
// GLSL declaration
uniform mat3 czm_viewRotation3D;
// Example
vec3 eyeVector = czm_viewRotation3D * worldVector;
debugShowBoundingVolume :Boolean
Draws the DrawCommand#boundingVolume
for this command, assuming it is a sphere, when the command executes.
Type:
- Boolean
- Default Value:
- false
- Source:
- See:
-
- DrawCommand#boundingVolume
enablePickFeatures :Boolean
WebMapServiceImageryProvider#pickFeatures
will
invoke the GetFeatureInfo
service on the WMS server and attempt to interpret the features included in the response. If false,
WebMapServiceImageryProvider#pickFeatures
will immediately return undefined (indicating no pickable
features) without communicating with the server. Set this property to false if you know your data
source does not support picking features or if you don't want this provider's features to be pickable.
Type:
- Boolean
- Default Value:
- true
- Source:
executeInClosestFrustum :Boolean
false
.
Type:
- Boolean
- Default Value:
- false
- Source:
framebuffer :Framebuffer
Type:
- Default Value:
- undefined
- Source:
instanceCount :Number
Type:
- Number
- Default Value:
- 0
- Source:
layers :String
Type:
- String
modelMatrix :Matrix4
When undefined
, the geometry is assumed to be defined in world space.
Type:
- Default Value:
- undefined
- Source:
offset :Number
Type:
- Number
- Default Value:
- 0
- Source:
orientedBoundingBox :OrientedBoundingBox
DrawCommand#boundingVolume
for plane intersection testing.
Type:
- Default Value:
- undefined
- Source:
- See:
-
- DrawCommand#debugShowBoundingVolume
owner :Object
Scene#debugCommandFilter
.
Type:
- Object
- Default Value:
- undefined
- Source:
- See:
pass :Pass
Type:
- Pass
- Default Value:
- undefined
- Source:
(readonly) pickFeaturesUrl :String
UrlTemplateImageryProvider#pickFeatures
will immediately returned undefined, indicating no
features picked. The URL template supports all of the keywords supported by the
UrlTemplateImageryProvider#url
property, plus the following:
{i}
: The pixel column (horizontal coordinate) of the picked position, where the Westernmost pixel is 0.{j}
: The pixel row (vertical coordinate) of the picked position, where the Northernmost pixel is 0.{reverseI}
: The pixel column (horizontal coordinate) of the picked position, where the Easternmost pixel is 0.{reverseJ}
: The pixel row (vertical coordinate) of the picked position, where the Southernmost pixel is 0.{longitudeDegrees}
: The longitude of the picked position in degrees.{latitudeDegrees}
: The latitude of the picked position in degrees.{longitudeProjected}
: The longitude of the picked position in the projected coordinates of the tiling scheme.{latitudeProjected}
: The latitude of the picked position in the projected coordinates of the tiling scheme.{format}
: The format in which to get feature information, as specified in theGetFeatureInfoFormat
.
Type:
- String
primitiveType :PrimitiveType
Type:
- PrimitiveType
- Default Value:
- PrimitiveType.TRIANGLES
- Source:
receiveShadows :Boolean
Type:
- Boolean
- Default Value:
- false
- Source:
removeOnStop :Boolean
true
, the animation is removed after it stops playing.
This is slightly more efficient that not removing it, but if, for example,
time is reversed, the animation is not played again.
Type:
- Boolean
- Default Value:
- false
- Source:
renderState :RenderState
Type:
- RenderState
- Default Value:
- undefined
- Source:
shaderProgram :ShaderProgram
Type:
- Default Value:
- undefined
- Source:
start :Event
This event is fired at the end of the frame after the scene is rendered.
Type:
- Default Value:
- new Event()
- Source:
Example
animation.start.addEventListener(function(model, animation) {
console.log('Animation started: ' + animation.name);
});
stop :Event
This event is fired at the end of the frame after the scene is rendered.
Type:
- Default Value:
- new Event()
- Source:
Example
animation.stop.addEventListener(function(model, animation) {
console.log('Animation stopped: ' + animation.name);
});
uniformMap :Object
Type:
- Object
- Default Value:
- undefined
- Source:
update :Event
This event is fired at the end of the frame after the scene is rendered.
Type:
- Default Value:
- new Event()
- Source:
Example
animation.update.addEventListener(function(model, animation, time) {
console.log('Animation updated: ' + animation.name + '. glTF animation time: ' + time);
});
vertexArray :VertexArray
Type:
- VertexArray
- Default Value:
- undefined
- Source:
Methods
Billboard()
BillboardCollection
. A billboard is created and its initial
properties are set by calling BillboardCollection#add
.
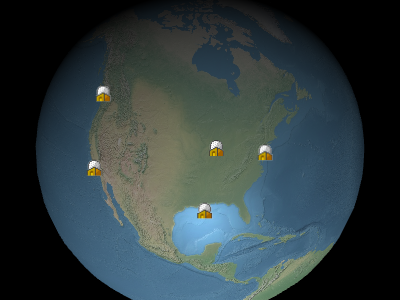
Example billboards
- Source:
- See:
Throws:
-
-
scaleByDistance.far must be greater than scaleByDistance.near
- Type
- DeveloperError
-
-
-
translucencyByDistance.far must be greater than translucencyByDistance.near
- Type
- DeveloperError
-
-
-
pixelOffsetScaleByDistance.far must be greater than pixelOffsetScaleByDistance.near
- Type
- DeveloperError
-
-
-
distanceDisplayCondition.far must be greater than distanceDisplayCondition.near
- Type
- DeveloperError
-
Label()
LabelCollection#add
.
- Source:
- See:
Throws:
-
-
translucencyByDistance.far must be greater than translucencyByDistance.near
- Type
- DeveloperError
-
-
-
pixelOffsetScaleByDistance.far must be greater than pixelOffsetScaleByDistance.near
- Type
- DeveloperError
-
-
-
distanceDisplayCondition.far must be greater than distanceDisplayCondition.near
- Type
- DeveloperError
-
ModelAnimation()
ModelAnimationCollection
. An active animation is an
instance of an animation; for example, there can be multiple active animations
for the same glTF animation, each with a different start time.
Create this by calling ModelAnimationCollection#add
.
- Source:
- See:
ModelAnimationCollection()
Model#activeAnimations
.
- Source:
- See:
ModelMaterial()
Use Model#getMaterial
to create an instance.
- Source:
- See:
ModelMesh()
Use Model#getMesh
to create an instance.
- Source:
- See:
ModelNode()
Use Model#getNode
to create an instance.
- Source:
- See:
Example
var node = model.getNode('LOD3sp');
node.matrix = Cesium.Matrix4.fromScale(new Cesium.Cartesian3(5.0, 1.0, 1.0), node.matrix);
PointPrimitive()
PointPrimitiveCollection
. A point is created and its initial
properties are set by calling PointPrimitiveCollection#add
.
Throws:
-
-
scaleByDistance.far must be greater than scaleByDistance.near
- Type
- DeveloperError
-
-
-
translucencyByDistance.far must be greater than translucencyByDistance.near
- Type
- DeveloperError
-
-
-
distanceDisplayCondition.far must be greater than distanceDisplayCondition.near
- Type
- DeveloperError
-
Polyline(optionsopt)
PolylineCollection#add
Parameters:
Name | Type | Attributes | Description | ||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
Object |
<optional> |
Object with the following properties:
Properties
|
- Source:
- See: