Tracing Reference¶
New in version 3.0.
A reference for client tracing API.
See also
Client Tracing for tracing usage instructions.
Request life cycle¶
A request goes through the following stages and corresponding fallbacks.
Overview¶

Name | Description |
---|---|
start | on_request_start |
redirect | on_request_redirect |
acquire_connection | Connection acquiring |
headers_received | |
exception | on_request_exception |
end | on_request_end |
headers_sent | |
chunk_sent | on_request_chunk_sent |
chunk_received | on_response_chunk_received |
Connection acquiring¶
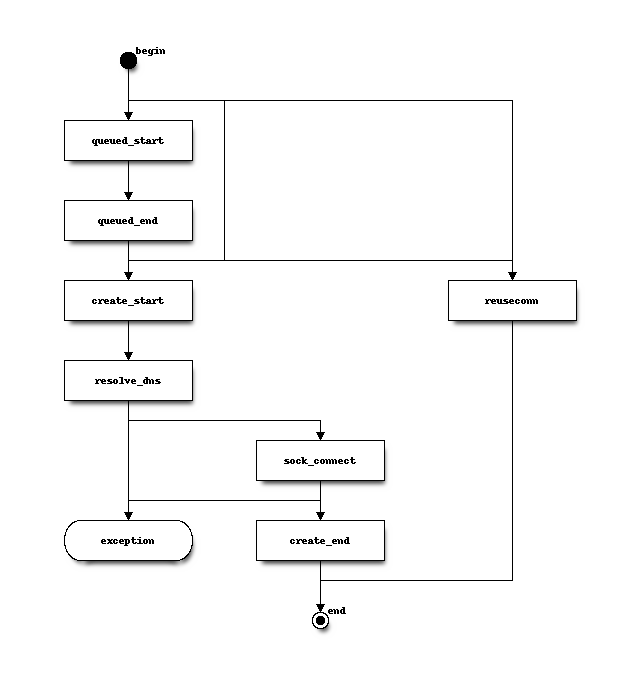
Name | Description |
---|---|
begin | |
end | |
queued_start | on_connection_queued_start |
create_start | on_connection_create_start |
reuseconn | on_connection_reuseconn |
queued_end | on_connection_queued_end |
create_end | on_connection_create_end |
exception | Exception raised |
resolve_dns | DNS resolving |
sock_connect | Connection establishment |
DNS resolving¶
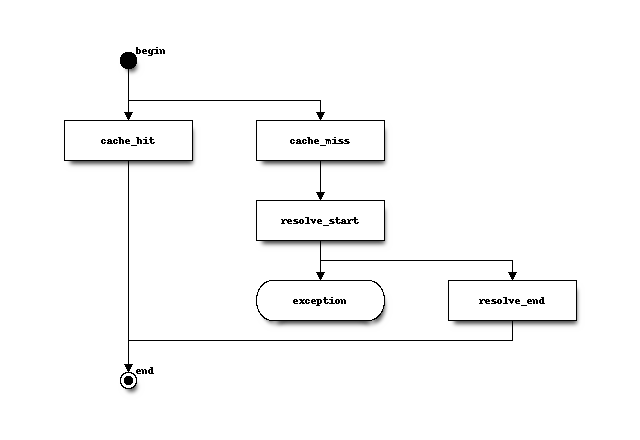
Name | Description |
---|---|
begin | |
end | |
exception | Exception raised |
resolve_end | on_dns_resolvehost_end |
resolve_start | on_dns_resolvehost_start |
cache_hit | on_dns_cache_hit |
cache_miss | on_dns_cache_miss |
TraceConfig¶
-
class
aiohttp.
TraceConfig
(trace_config_ctx_factory=SimpleNamespace)¶ Trace config is the configuration object used to trace requests launched by a
ClientSession
object using different events related to different parts of the request flow.Parameters: trace_config_ctx_factory – factory used to create trace contexts, default class used types.SimpleNamespace
-
trace_config_ctx
(trace_request_ctx=None)¶ Parameters: trace_request_ctx – Will be used to pass as a kw for the trace_config_ctx_factory
.Build a new trace context from the config.
Every signal handler should have the following signature:
async def on_signal(session, context, params): ...
where
session
isClientSession
instance,context
is an object returned bytrace_config_ctx()
call andparams
is a data class with signal parameters. The type ofparams
depends on subscribed signal and described below.-
on_request_start
¶ Property that gives access to the signals that will be executed when a request starts.
params
isaiohttp.TraceRequestStartParams
instance.
-
on_request_chunk_sent
¶ Property that gives access to the signals that will be executed when a chunk of request body is sent.
params
isaiohttp.TraceRequestChunkSentParams
instance.New in version 3.1.
-
on_response_chunk_received
¶ Property that gives access to the signals that will be executed when a chunk of response body is received.
params
isaiohttp.TraceResponseChunkReceivedParams
instance.New in version 3.1.
-
on_request_redirect
¶ Property that gives access to the signals that will be executed when a redirect happens during a request flow.
params
isaiohttp.TraceRequestRedirectParams
instance.
-
on_request_end
¶ Property that gives access to the signals that will be executed when a request ends.
params
isaiohttp.TraceRequestEndParams
instance.
-
on_request_exception
¶ Property that gives access to the signals that will be executed when a request finishes with an exception.
params
isaiohttp.TraceRequestExceptionParams
instance.
-
on_connection_queued_start
¶ Property that gives access to the signals that will be executed when a request has been queued waiting for an available connection.
params
isaiohttp.TraceConnectionQueuedStartParams
instance.
-
on_connection_queued_end
¶ Property that gives access to the signals that will be executed when a request that was queued already has an available connection.
params
isaiohttp.TraceConnectionQueuedEndParams
instance.
-
on_connection_create_start
¶ Property that gives access to the signals that will be executed when a request creates a new connection.
params
isaiohttp.TraceConnectionCreateStartParams
instance.
-
on_connection_create_end
¶ Property that gives access to the signals that will be executed when a request that created a new connection finishes its creation.
params
isaiohttp.TraceConnectionCreateEndParams
instance.
-
on_connection_reuseconn
¶ Property that gives access to the signals that will be executed when a request reuses a connection.
params
isaiohttp.TraceConnectionReuseconnParams
instance.
-
on_dns_resolvehost_start
¶ Property that gives access to the signals that will be executed when a request starts to resolve the domain related with the request.
params
isaiohttp.TraceDnsResolveHostStartParams
instance.
-
on_dns_resolvehost_end
¶ Property that gives access to the signals that will be executed when a request finishes to resolve the domain related with the request.
params
isaiohttp.TraceDnsResolveHostEndParams
instance.
-
on_dns_cache_hit
¶ Property that gives access to the signals that will be executed when a request was able to use a cached DNS resolution for the domain related with the request.
params
isaiohttp.TraceDnsCacheHitParams
instance.
-
on_dns_cache_miss
¶ Property that gives access to the signals that will be executed when a request was not able to use a cached DNS resolution for the domain related with the request.
params
isaiohttp.TraceDnsCacheMissParams
instance.
-
TraceRequestStartParams¶
-
class
aiohttp.
TraceRequestStartParams
¶ See
TraceConfig.on_request_start
for details.-
method
¶ Method that will be used to make the request.
-
url
¶ URL that will be used for the request.
-
headers
¶ Headers that will be used for the request, can be mutated.
-
TraceRequestChunkSentParams¶
-
class
aiohttp.
TraceRequestChunkSentParams
¶ New in version 3.1.
See
TraceConfig.on_request_chunk_sent
for details.-
chunk
¶ Bytes of chunk sent
-
TraceResponseChunkSentParams¶
-
class
aiohttp.
TraceResponseChunkSentParams
¶ New in version 3.1.
See
TraceConfig.on_response_chunk_received
for details.-
chunk
¶ Bytes of chunk received
-
TraceRequestEndParams¶
-
class
aiohttp.
TraceRequestEndParams
¶ See
TraceConfig.on_request_end
for details.-
method
¶ Method used to make the request.
-
url
¶ URL used for the request.
-
headers
¶ Headers used for the request.
-
response
¶ Response
ClientResponse
.
-
TraceRequestExceptionParams¶
TraceRequestRedirectParams¶
-
class
aiohttp.
TraceRequestRedirectParams
¶ See
TraceConfig.on_request_redirect
for details.-
method
¶ Method used to get this redirect request.
-
url
¶ URL used for this redirect request.
-
headers
¶ Headers used for this redirect.
-
response
¶ Response
ClientResponse
got from the redirect.
-
TraceConnectionQueuedStartParams¶
-
class
aiohttp.
TraceConnectionQueuedStartParams
¶ See
TraceConfig.on_connection_queued_start
for details.There are no attributes right now.
TraceConnectionQueuedEndParams¶
-
class
aiohttp.
TraceConnectionQueuedEndParams
¶ See
TraceConfig.on_connection_queued_end
for details.There are no attributes right now.
TraceConnectionCreateStartParams¶
-
class
aiohttp.
TraceConnectionCreateStartParams
¶ See
TraceConfig.on_connection_create_start
for details.There are no attributes right now.
TraceConnectionCreateEndParams¶
-
class
aiohttp.
TraceConnectionCreateEndParams
¶ See
TraceConfig.on_connection_create_end
for details.There are no attributes right now.
TraceConnectionReuseconnParams¶
-
class
aiohttp.
TraceConnectionReuseconnParams
¶ See
TraceConfig.on_connection_reuseconn
for details.There are no attributes right now.
TraceDnsResolveHostStartParams¶
-
class
aiohttp.
TraceDnsResolveHostStartParams
¶ See
TraceConfig.on_dns_resolvehost_start
for details.-
Host
¶ Host that will be resolved.
-
TraceDnsResolveHostEndParams¶
-
class
aiohttp.
TraceDnsResolveHostEndParams
¶ See
TraceConfig.on_dns_resolvehost_end
for details.-
Host
¶ Host that has been resolved.
-
TraceDnsCacheHitParams¶
-
class
aiohttp.
TraceDnsCacheHitParams
¶ See
TraceConfig.on_dns_cache_hit
for details.-
Host
¶ Host found in the cache.
-
TraceDnsCacheMissParams¶
-
class
aiohttp.
TraceDnsCacheMissParams
¶ See
TraceConfig.on_dns_cache_miss
for details.-
Host
¶ Host didn’t find the cache.
-